지난 1탄에서는 content-type이 application/json
일때 문서 작성하는 법에 대해 다뤄보았다. 이번에는 x-www-form-urlencoded
형식의 api 일때 작성하는 법에 대해 다뤄보자.
기본적인 환경설정은 동일하기 때문에 패스하고
- 테스트 코드 작성시 사용하는 메소드
- index.adoc 설정법
만 다르기 때문에 이것만 다루도록 하겠다.
[1] 테스트코드 작성하기
1. 테스트 대상 코드
x-www-form-urlencoded 방식으로 전달 시 메소드는 POST 로 전달된다. 아래와 같이 테스트할 api를 작성해 준다.
@PostMapping("/tmp2")
fun tmp2(requestDto: TmpRequestDto): TmpResponseDto {
val recommendEmail = requestDto.name + "@gmail.com"
val responseDto =
TmpResponseDto(
code = 200,
email = recommendEmail,
message = "hello, ${requestDto.nickname ?: "guest"}",
)
return responseDto
}
2. 테스트코드 : params
application/json 타입일때에는 요청 파라미터를 정의할때 requestFields 메소드를 사용하였다. 하지만 x-www-form-urlencoded일때에는 params를 사용해야 한다.
// given
val requestDto =
HealthcheckController.TmpRequestDto(
userId = 1,
name = "tester2",
nickname = "banana",
age = 25,
productName = "잘팔리는노트",
hobby = mutableListOf("클라이밍", "뜨개질"),
address =
HealthcheckController.TmpAddress(
city = "세종특별시 아름동",
street = "654-321",
zipcode = 897,
),
)
val xxxRequest =
LinkedMultiValueMap(
mapOf(
"userId" to listOf(requestDto.userId.toString()),
"name" to listOf(requestDto.name),
"nickname" to listOf(requestDto.nickname),
"age" to listOf(requestDto.age.toString()),
"productName" to listOf(requestDto.productName),
"hobby" to listOf(requestDto.hobby.joinToString(",")),
"address.city" to listOf(requestDto.address.city),
"address.street" to listOf(requestDto.address.street),
"address.zipcode" to listOf(requestDto.address.zipcode.toString()),
),
)
+) Map<String!, String!>
params의 value 타입에 맞춰 작성해 주어야 한다. 이때 문제가 될만한 것으로는 list 타입의 hobby이다.
콤마 (,) 로 구분하여 string으로 변환하는 작업을 해준 뒤 params의 값으로 작성해야 한다.
// when
val result =
this.mockMvc.perform(
RestDocumentationRequestBuilders
.post("$domain/tmp2")
.contentType(MediaType.APPLICATION_FORM_URLENCODED)
.params(xxxRequest)
.accept(MediaType.APPLICATION_JSON),
)
이제 요청 준비는 다 되었고 결과값을 받아 문서로 만들 코드를 작성한다.
// then
result
.andExpect(MockMvcResultMatchers.status().isOk)
.andDo(
document(
"docs_xxxform",
documentRequest,
documentResponse,
formParameters(
parameterWithName("userId").description("유저Id").attributes(Attributes.key("type").value("integer")),
parameterWithName("name").description("이름").attributes(Attributes.key("type").value("string")),
parameterWithName("nickname").description("닉네임").attributes(Attributes.key("type").value("string")),
parameterWithName("age").description("나이").attributes(Attributes.key("type").value("integer")),
parameterWithName("productName").description("상품명").attributes(Attributes.key("type").value("string")),
parameterWithName("hobby").description("취미").attributes(Attributes.key("type").value("array")),
parameterWithName("address.city").description("도시").attributes(Attributes.key("type").value("string")),
parameterWithName("address.street").description("도로명").attributes(Attributes.key("type").value("string")),
parameterWithName("address.zipcode").description("우편번호").attributes(Attributes.key("type").value("integer")),
),
responseFields(
fieldWithPath("code").description("code").optional(),
fieldWithPath("email").description("이메일").optional(),
fieldWithPath("message").description("메시지").optional(),
),
),
)
+) formParameters
요청파라미터를 정의하는 메소드로 formParameters
를 사용한다. 객체안의 키는 application/json 형식때와 같이 마침표로 구분하여 작성한다.
[2] 문서 생성하기
1. 테스트 실행 : adoc 파일 생성
테스트를 실행하여 docs_xxxform
에 대한 adoc 파일들이 생성되는걸 확인해보자
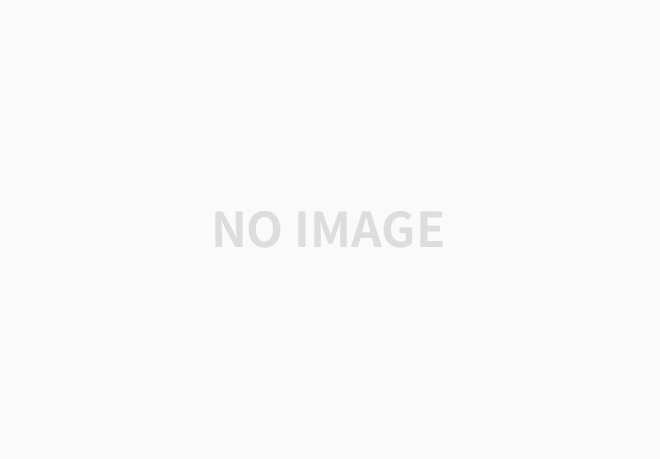
이전에 생성된 docs_json
과 함께 테스트 성공으로 docs-xxxform
폴더가 생성되었다.
2. rest docs 포맷 설정 : index.adoc
x-www-form-urlencoded
형식에 맞춰 Index.adoc 폼도 수정해 주어야 한다.
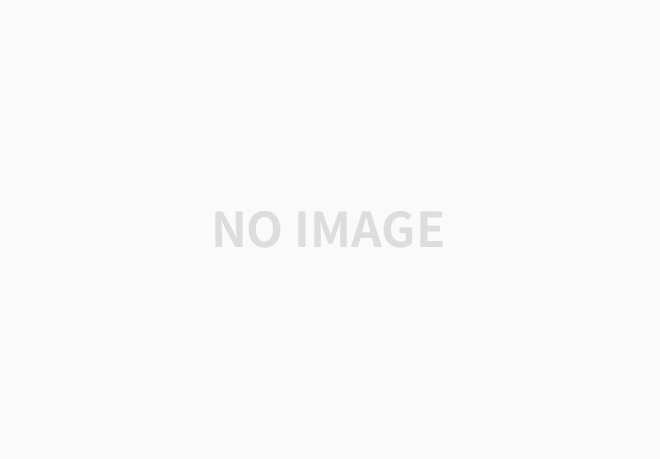
application/json과 x-www-form-urlencdoed의 차이점!
- request-fields.adoc
은 application/json 에만 있다
- urlencoded에는 form-parameters.adoc
이 있다.
위 두가지가 요청 필드에 대한 명세서가 되므로 이에 유의하여 index.adoc파일을 작성해 준다.
# REST API
## [1] REST DOCS EXAMPLE : application/json
### [http : 요청]
include::{snippets}/docs_json/http-request.adoc[]
#### [요청 필드]
include::{snippets}/docs_json/request-fields.adoc[]
### [http : 응답]
include::{snippets}/docs_json/http-response.adoc[]
#### [응답 필드]
include::{snippets}/docs_json/response-fields.adoc[]
## [2] REST DOCS EXAMPLE : x-www-form-urlendcoded
### [http : 요청]
include::{snippets}/docs_xxxform/http-request.adoc[]
#### [요청 필드]
include::{snippets}/docs_xxxform/form-parameters.adoc[]
### [http : 응답]
include::{snippets}/docs_xxxform/http-response.adoc[]
#### [응답 필드]
include::{snippets}/docs_xxxform/response-fields.adoc[]
이제 build를 하고 docs/index.html
경로로 만들어진 문서를 확인해보자
3. /docs/index.html 로 발행된 문서 확인
참고로! 테스트 실행을 하고나서 build 하지 않고, build 시 테스트코드가 자동으로 실행되는 설정이라면 바로 build만 해도된다.
또 로컬환경에서 실행중일때 어라?! 빌드 됐는데 왜 문서 안바뀌지?! 하지 말고 서버도 재실행 해주는걸 잊지말자 🙂
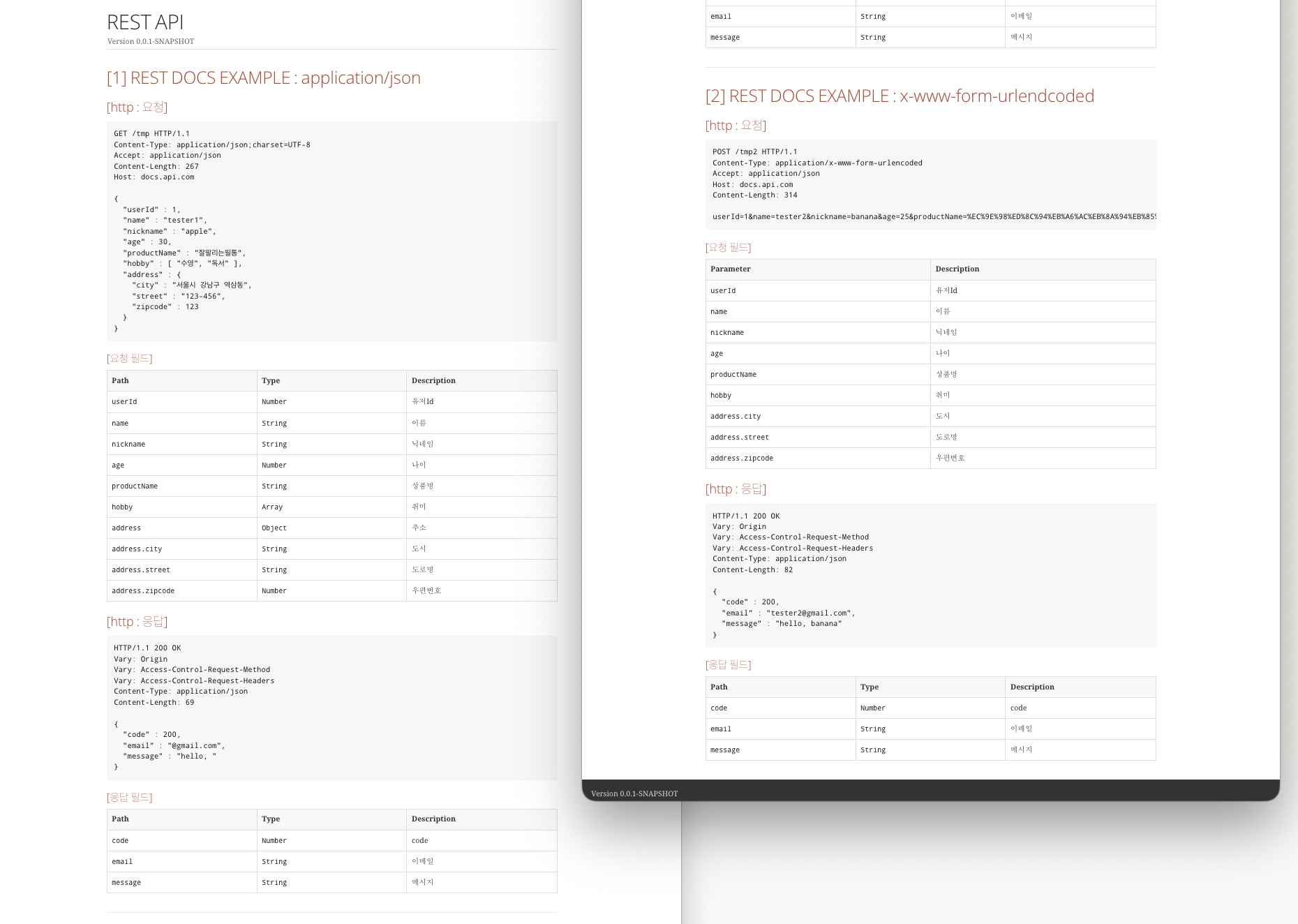
한 페이지에 작성되다 보니 너무 길어서 창 두개에 띄움!
이렇게 content-type이 x-www-form-urlencoded
일때에 spring rest docs 작성도 완성했다! 다음은 이 문서를 보기좋게 커스텀 하는 방법에 대해 다뤄보고자 한다.
'DEV-ing log > Spring' 카테고리의 다른 글
[Kotlin / Spring] Spring Rest Docs 1탄 - 시작하기 : application/json (0) | 2024.09.12 |
---|---|
[Spring | H2] h2 테이블 생성 안되는 오류 ⚒️ : 예약어 문제 (0) | 2024.04.30 |
[IntelliJ] Live Template 에 커스텀 단축키 생성하기 (1) | 2024.04.28 |
[스프링 프로젝트] 초기 세팅시 확인할 것 뽀인트 (0) | 2024.04.26 |
[스프링 핵심원리 - 기본편] 메모 (0) | 2024.04.22 |